Ensemble studying methods primarily fall into two classes: bagging and boosting. Bagging improves stability and accuracy by aggregating unbiased predictions, whereas boosting sequentially corrects the errors of prior fashions, enhancing their efficiency with every iteration. This publish begins our deep dive into boosting, beginning with the Gradient Boosting Regressor. By way of its utility on the Ames Housing Dataset, we are going to display how boosting uniquely enhances fashions, setting the stage for exploring numerous boosting methods in upcoming posts.
Let’s get began.
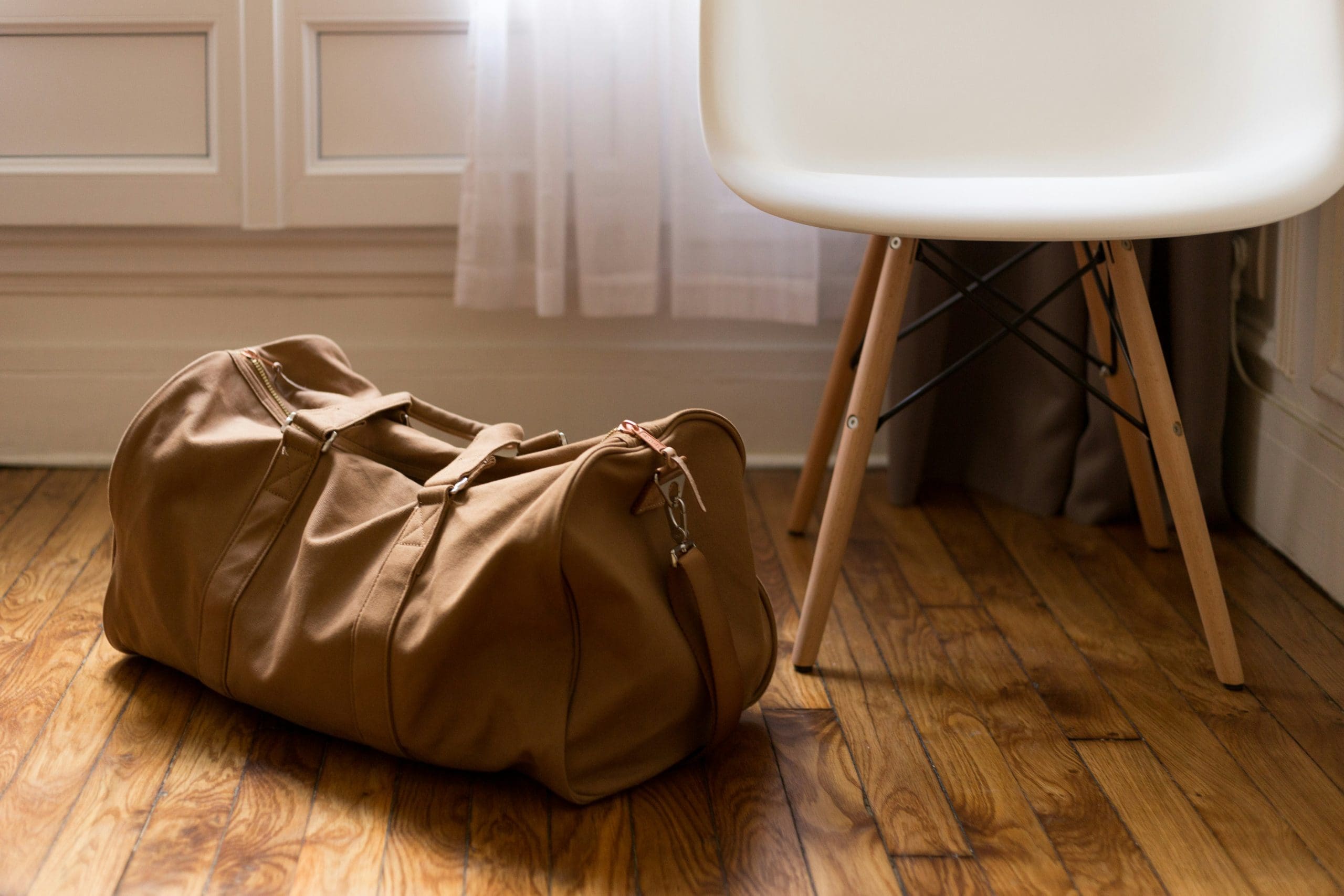
Boosting Over Bagging: Enhancing Predictive Accuracy with Gradient Boosting Regressors
Photograph by Erol Ahmed. Some rights reserved.
Overview
This publish is split into 4 elements; they’re:
- What’s Boosting?
- Evaluating Mannequin Efficiency: Choice Tree Baseline to Gradient Boosting Ensembles
- Optimizing Gradient Boosting with Studying Charge Changes
- Closing Optimization: Tuning Studying Charge and Variety of Timber
What’s Boosting?
Boosting is an ensemble approach combining a number of fashions to create a powerful learner. In contrast to different ensemble strategies that will construct fashions in parallel, boosting provides fashions sequentially, with every new mannequin specializing in enhancing the areas the place earlier fashions struggled. This methodically improves the ensemble’s accuracy with every iteration, making it notably efficient for complicated datasets.
Key Options of Boosting:
- Sequential Studying: Boosting builds one mannequin at a time. Every new mannequin learns from the shortcomings of the earlier ones, permitting for progressive enchancment in capturing information complexities.
- Error Correction: New learners deal with beforehand mispredicted situations, constantly enhancing the ensemble’s functionality to seize tough patterns within the information.
- Mannequin Complexity: The ensemble’s complexity grows as extra fashions are added, enabling it to seize intricate information constructions successfully.
Boosting vs. Bagging
Bagging includes constructing a number of fashions (usually independently) and mixing their outputs to reinforce the ensemble’s total efficiency, primarily by lowering the danger of overfitting the noise within the coaching information, in distinction, boosting focuses on enhancing the accuracy of predictions by studying from errors sequentially, which permits it to adapt extra intricately to the info.
Boosting Regressors in scikit-learn:
Scikit-learn supplies a number of implementations of boosting, tailor-made for various wants and information situations:
- AdaBoost Regressor: Employs a sequence of weak learners and adjusts their focus primarily based on the errors of the earlier mannequin, enhancing the place previous fashions had been missing.
- Gradient Boosting Regressor: Builds fashions one by one, with every new mannequin educated to appropriate the residuals (errors) made by the earlier ones, enhancing accuracy by way of cautious changes.
- HistGradient Boosting Regressor: An optimized type of Gradient Boosting designed for bigger datasets, which hastens calculations by utilizing histograms to approximate gradients.
Every methodology makes use of the core rules of boosting to enhance its parts’ efficiency, showcasing the flexibility and energy of this method in tackling predictive modeling challenges. Within the following sections of this publish, we are going to display a sensible utility of the Gradient Boosting Regressor utilizing the Ames Housing Dataset.
Evaluating Mannequin Efficiency: Choice Tree Baseline to Gradient Boosting Ensembles
In transitioning from the theoretical elements of boosting to its sensible functions, this part will display the Gradient Boosting Regressor utilizing the meticulously preprocessed Ames Housing Dataset. Our preprocessing steps, constant throughout numerous tree-based fashions, be certain that the enhancements noticed could be attributed on to the mannequin’s capabilities, setting the stage for an efficient comparability.
The code under establishes our comparative evaluation framework by first organising a baseline utilizing a single Choice Tree, which isn’t an ensemble methodology. This baseline will permit us as an instance the incremental advantages introduced by precise ensemble strategies clearly. Following this, we configure two variations, every of Bagging, Random Forest, and the Gradient Boosting Regressor, with 100 and 200 timber, respectively, to discover the enhancements these ensemble methods provide over the baseline.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 |
# Import essential libraries for preprocessing and modeling import pandas as pd from sklearn.pipeline import Pipeline from sklearn.impute import SimpleImputer from sklearn.compose import ColumnTransformer from sklearn.tree import DecisionTreeRegressor from sklearn.model_selection import cross_val_score from sklearn.preprocessing import OrdinalEncoder, OneHotEncoder, FunctionTransformer from sklearn.ensemble import GradientBoostingRegressor, BaggingRegressor, RandomForestRegressor
# Load the dataset Ames = pd.read_csv(‘Ames.csv’)
# Regulate information varieties for categorical variables for col in [‘MSSubClass’, ‘YrSold’, ‘MoSold’]: Ames[col] = Ames[col].astype(‘object’)
# Exclude ‘PID’ and ‘SalePrice’ from options and particularly deal with the ‘Electrical’ column numeric_features = Ames.select_dtypes(embody=[‘int64’, ‘float64’]).drop(columns=[‘PID’, ‘SalePrice’]).columns categorical_features = Ames.select_dtypes(embody=[‘object’]).columns.distinction([‘Electrical’]) electrical_feature = [‘Electrical’]
# Manually specify the classes for ordinal encoding in line with the info dictionary ordinal_order = { ‘Electrical’: [‘Mix’, ‘FuseP’, ‘FuseF’, ‘FuseA’, ‘SBrkr’], # Electrical system ‘LotShape’: [‘IR3’, ‘IR2’, ‘IR1’, ‘Reg’], # Common form of property ‘Utilities’: [‘ELO’, ‘NoSeWa’, ‘NoSewr’, ‘AllPub’], # Sort of utilities accessible ‘LandSlope’: [‘Sev’, ‘Mod’, ‘Gtl’], # Slope of property ‘ExterQual’: [‘Po’, ‘Fa’, ‘TA’, ‘Gd’, ‘Ex’], # Evaluates the standard of the fabric on the outside ‘ExterCond’: [‘Po’, ‘Fa’, ‘TA’, ‘Gd’, ‘Ex’], # Evaluates the current situation of the fabric on the outside ‘BsmtQual’: [‘None’, ‘Po’, ‘Fa’, ‘TA’, ‘Gd’, ‘Ex’], # Top of the basement ‘BsmtCond’: [‘None’, ‘Po’, ‘Fa’, ‘TA’, ‘Gd’, ‘Ex’], # Common situation of the basement ‘BsmtExposure’: [‘None’, ‘No’, ‘Mn’, ‘Av’, ‘Gd’], # Walkout or backyard degree basement partitions ‘BsmtFinType1’: [‘None’, ‘Unf’, ‘LwQ’, ‘Rec’, ‘BLQ’, ‘ALQ’, ‘GLQ’], # High quality of basement completed space ‘BsmtFinType2’: [‘None’, ‘Unf’, ‘LwQ’, ‘Rec’, ‘BLQ’, ‘ALQ’, ‘GLQ’], # High quality of second basement completed space ‘HeatingQC’: [‘Po’, ‘Fa’, ‘TA’, ‘Gd’, ‘Ex’], # Heating high quality and situation ‘KitchenQual’: [‘Po’, ‘Fa’, ‘TA’, ‘Gd’, ‘Ex’], # Kitchen high quality ‘Useful’: [‘Sal’, ‘Sev’, ‘Maj2’, ‘Maj1’, ‘Mod’, ‘Min2’, ‘Min1’, ‘Typ’], # House performance ‘FireplaceQu’: [‘None’, ‘Po’, ‘Fa’, ‘TA’, ‘Gd’, ‘Ex’], # Fire high quality ‘GarageFinish’: [‘None’, ‘Unf’, ‘RFn’, ‘Fin’], # Inside end of the storage ‘GarageQual’: [‘None’, ‘Po’, ‘Fa’, ‘TA’, ‘Gd’, ‘Ex’], # Storage high quality ‘GarageCond’: [‘None’, ‘Po’, ‘Fa’, ‘TA’, ‘Gd’, ‘Ex’], # Storage situation ‘PavedDrive’: [‘N’, ‘P’, ‘Y’], # Paved driveway ‘PoolQC’: [‘None’, ‘Fa’, ‘TA’, ‘Gd’, ‘Ex’], # Pool high quality ‘Fence’: [‘None’, ‘MnWw’, ‘GdWo’, ‘MnPrv’, ‘GdPrv’] # Fence high quality }
# Extract listing of ALL ordinal options from dictionary ordinal_features = listing(ordinal_order.keys())
# Checklist of ordinal options besides Electrical ordinal_except_electrical = [feature for feature in ordinal_features if feature != ‘Electrical’]
# Outline transformations for numerous function varieties electrical_transformer = Pipeline(steps=[ (‘impute_electrical’, SimpleImputer(strategy=‘most_frequent’)), (‘ordinal_electrical’, OrdinalEncoder(categories=[ordinal_order[‘Electrical’]])) ])
numeric_transformer = Pipeline(steps=[ (‘impute_mean’, SimpleImputer(strategy=‘mean’)) ])
# Up to date categorical imputer utilizing SimpleImputer categorical_imputer = SimpleImputer(technique=‘fixed’, fill_value=‘None’)
ordinal_transformer = Pipeline([ (‘impute_ordinal’, categorical_imputer), (‘ordinal’, OrdinalEncoder(categories=[ordinal_order[feature] for function in ordinal_features if function in ordinal_except_electrical])) ])
nominal_features = [feature for feature in categorical_features if feature not in ordinal_features] categorical_transformer = Pipeline([ (‘impute_nominal’, categorical_imputer), (‘onehot’, OneHotEncoder(handle_unknown=‘ignore’)) ])
# Mixed preprocessor for numeric, ordinal, nominal, and particular electrical information preprocessor = ColumnTransformer( transformers=[ (‘electrical’, electrical_transformer, [‘Electrical’]), (‘num’, numeric_transformer, numeric_features), (‘ordinal’, ordinal_transformer, ordinal_except_electrical), (‘nominal’, categorical_transformer, nominal_features) ])
# Outline mannequin pipelines together with Gradient Boosting Regressor fashions = { ‘Choice Tree (1 Tree)’: DecisionTreeRegressor(random_state=42), ‘Bagging Regressor (100 Choice Timber)’: BaggingRegressor(base_estimator=DecisionTreeRegressor(random_state=42), n_estimators=100, random_state=42), ‘Bagging Regressor (200 Choice Timber)’: BaggingRegressor(base_estimator=DecisionTreeRegressor(random_state=42), n_estimators=200, random_state=42), ‘Random Forest (Default of 100 Timber)’: RandomForestRegressor(random_state=42), ‘Random Forest (200 Timber)’: RandomForestRegressor(n_estimators=200, random_state=42), ‘Gradient Boosting Regressor (Default of 100 Timber)’: GradientBoostingRegressor(random_state=42), ‘Gradient Boosting Regressor (200 Timber)’: GradientBoostingRegressor(n_estimators=200, random_state=42) }
# Consider fashions utilizing cross-validation and print outcomes outcomes = {} for identify, mannequin in fashions.gadgets(): model_pipeline = Pipeline([ (‘preprocessor’, preprocessor), (‘regressor’, model) ]) scores = cross_val_score(model_pipeline, Ames.drop(columns=‘SalePrice’), Ames[‘SalePrice’], cv=5) outcomes[name] = spherical(scores.imply(), 4) print(f“{identify}: Imply CV R² = {outcomes[name]}”) |
Beneath are the cross-validation outcomes, showcasing how every mannequin performs when it comes to imply R² values:
Choice Tree (1 Tree): Imply CV R² = 0.7663 Bagging Regressor (100 Choice Timber): Imply CV R² = 0.8957 Bagging Regressor (200 Choice Timber): Imply CV R² = 0.897 Random Forest (Default of 100 Timber): Imply CV R² = 0.8954 Random Forest (200 Timber): Imply CV R² = 0.8969 Gradient Boosting Regressor (Default of 100 Timber): Imply CV R² = 0.9027 Gradient Boosting Regressor (200 Timber): Imply CV R² = 0.9061 |
The outcomes from our ensemble fashions underline a number of key insights into the habits and efficiency of superior regression methods:
- Baseline and Enhancement: Beginning with a fundamental Choice Tree Regressor, which serves as our baseline with an R² of 0.7663, we observe important efficiency uplifts as we introduce extra complicated fashions. Each Bagging and Random Forest Regressors, utilizing totally different numbers of timber, present improved scores, illustrating the ability of ensemble strategies in leveraging a number of studying fashions to cut back error.
- Gradient Boosting Regressor’s Edge: Notably notable is the Gradient Boosting Regressor. With its default setting of 100 timber, it achieves an R² of 0.9027, and additional growing the variety of timber to 200 nudges the rating as much as 0.9061. This means the effectiveness of GBR on this context and highlights its effectivity in sequential enchancment from further learners.
- Marginal Positive factors from Extra Timber: Whereas growing the variety of timber usually ends in higher efficiency, the incremental positive factors diminish as we develop the ensemble measurement. This pattern is clear throughout Bagging, Random Forest, and Gradient Boosting fashions, suggesting a degree of diminishing returns the place further computational sources yield minimal efficiency enhancements.
The outcomes spotlight the Gradient Boosting Regressor’s strong efficiency. It successfully leverages complete preprocessing and the sequential enchancment technique attribute of boosting. Subsequent, we are going to discover how adjusting the training price can refine our mannequin’s efficiency, enhancing its predictive accuracy.
Optimizing Gradient Boosting with Studying Charge Changes
The learning_rate
is exclusive to boosting fashions just like the Gradient Boosting Regressor, distinguishing it from different fashions resembling Choice Timber and Random Forests, which do not need a direct equal of this parameter. Adjusting the learning_rate
permits us to delve deeper into the mechanics of boosting and improve our mannequin’s predictive energy by fine-tuning how aggressively it learns from every successive tree.
What’s the Studying Charge?
Within the context of Gradient Boosting Regressors and different gradient descent-based algorithms, the “studying price” is a vital hyperparameter that controls the velocity at which the mannequin learns. At its core, the training price influences the scale of the steps the mannequin takes towards the optimum resolution throughout coaching. Right here’s a breakdown:
- Measurement of Steps: The educational price determines the magnitude of the updates to the mannequin’s weights throughout coaching. A better studying price makes bigger updates, permitting the mannequin to be taught quicker however on the threat of overshooting the optimum resolution. Conversely, a decrease studying price makes smaller updates, which implies the mannequin learns slower however with doubtlessly greater precision.
- Impression on Mannequin Coaching:
- Convergence: A studying price that’s too excessive might trigger the coaching course of to converge too rapidly to a suboptimal resolution, or it may not converge in any respect because it overshoots the minimal.
- Accuracy and Overfitting: A studying price that’s too low can lead the mannequin to be taught too slowly, which can require extra timber to realize related accuracy, doubtlessly resulting in overfitting if not monitored.
- Tuning: Selecting the best studying price balances velocity and accuracy. It’s usually chosen by way of trial and error or extra systematic approaches like GridSearchCV and RandomizedSearchCV, as adjusting the training price can considerably have an effect on the mannequin’s efficiency and coaching time.
By adjusting the training price, information scientists can management how rapidly a boosting mannequin adapts to the complexity of its errors. This makes the training price a strong software in fine-tuning mannequin efficiency, particularly in boosting algorithms the place every new tree is constructed to appropriate the residuals (errors) left by the earlier timber.
To optimize the learning_rate
, we begin with GridSearchCV, a scientific methodology that can discover predefined values ([0.001, 0.01, 0.1, 0.2, 0.3]) to determine the best setting for enhancing our mannequin’s accuracy.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
# Experiment with GridSearchCV from sklearn.model_selection import GridSearchCV
# Parameter grid for GridSearchCV param_grid = { ‘regressor__learning_rate’: [0.001, 0.01, 0.1, 0.2, 0.3] }
# Setup the GridSearchCV grid_search = GridSearchCV(model_pipeline, param_grid, cv=5, scoring=‘r2’, verbose=1)
# Match the GridSearchCV to the info grid_search.match(Ames.drop(columns=‘SalePrice’), Ames[‘SalePrice’])
# Finest parameters and greatest rating from Grid Search print(“Finest parameters (Grid Search):”, grid_search.best_params_) print(“Finest rating (Grid Search):”, spherical(grid_search.best_score_, 4)) |
Listed here are the outcomes from our GridSearchCV, centered solely on optimizing the learning_rate
parameter:
Becoming 5 folds for every of 5 candidates, totalling 25 matches Finest parameters (Grid Search): {‘regressor__learning_rate’: 0.1} Finest rating (Grid Search): 0.9061 |
Utilizing GridSearchCV, we discovered {that a} learning_rate
of 0.1 yielded the very best consequence, matching the default setting. This means that for our dataset and preprocessing setup, growing or lowering the speed round this worth doesn’t considerably enhance the mannequin.
Following this, we make the most of RandomizedSearchCV to develop our search. In contrast to GridSearchCV, RandomizedSearchCV randomly selects from a steady vary, permitting for a doubtlessly extra exact optimization by exploring between the usual values, thus offering a complete understanding of how delicate variations in learning_rate
can affect efficiency.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
# Experiment with RandomizedSearchCV from sklearn.model_selection import RandomizedSearchCV from scipy.stats import uniform
# Parameter distribution for RandomizedSearchCV param_dist = { ‘regressor__learning_rate’: uniform(0.001, 0.299) # Uniform distribution between 0.001 and 0.3 }
# Setup the RandomizedSearchCV random_search = RandomizedSearchCV(model_pipeline, param_distributions=param_dist, n_iter=50, cv=5, scoring=‘r2’, verbose=1, random_state=42)
# Match the RandomizedSearchCV to the info random_search.match(Ames.drop(columns=‘SalePrice’), Ames[‘SalePrice’])
# Finest parameters and greatest rating from Random Search print(“Finest parameters (Random Search):”, random_search.best_params_) print(“Finest rating (Random Search):”, spherical(random_search.best_score_, 4)) |
Contrasting with GridSearchCV, RandomizedSearchCV recognized a barely totally different optimum learning_rate
of roughly 0.158, which enhanced our mannequin’s efficiency. This enchancment underscores the worth of a randomized search, notably when fine-tuning fashions, as it could actually discover a extra numerous set of potentialities and doubtlessly yield higher configurations.
Becoming 5 folds for every of fifty candidates, totalling 250 matches Finest parameters (Random Search): {‘regressor__learning_rate’: 0.1579021730580391} Finest rating (Random Search): 0.9134 |
The optimization by way of RandomizedSearchCV has demonstrated its efficacy by pinpointing a studying price that pushes our mannequin’s efficiency to new heights, attaining an R² rating of 0.9134. These experiments with learning_rate
changes by way of GridSearchCV and RandomizedSearchCV illustrate the fragile steadiness required in tuning gradient boosting fashions. Additionally they spotlight the advantages of exploring each systematic and randomized parameter search methods to optimize a mannequin absolutely.
Inspired by the positive factors achieved by way of these optimization methods, we are going to now lengthen our focus to fine-tuning each the learning_rate
and n_estimators
concurrently. This subsequent section goals to uncover much more optimum settings by exploring the mixed affect of those essential parameters on our Gradient Boosting Regressor’s efficiency.
Closing Optimization: Tuning Studying Charge and Variety of Timber
We start with GridSearchCV to systematically discover combos of learning_rate
and n_estimators
. This method supplies a structured option to assess the affect of various each parameters on our mannequin’s accuracy.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
# Construct on earlier blocks of code # ‘preprocessor’ is already arrange as your preprocessing pipeline model_pipeline = Pipeline([ (‘preprocessor’, preprocessor), (‘regressor’, GradientBoostingRegressor(random_state=42)) ])
# Parameter grid for GridSearchCV param_grid = { ‘regressor__learning_rate’: [0.001, 0.01, 0.1, 0.2, 0.3], ‘regressor__n_estimators’: [100, 200, 300, 400, 500] }
# Setup the GridSearchCV grid_search = GridSearchCV(model_pipeline, param_grid, cv=5, scoring=‘r2’, verbose=1)
# Match the GridSearchCV to the info grid_search.match(Ames.drop(columns=‘SalePrice’), Ames[‘SalePrice’])
# Finest parameters and greatest rating from Grid Search print(“Finest parameters (Grid Search):”, grid_search.best_params_) print(“Finest rating (Grid Search):”, spherical((grid_search.best_score_), 4)) |
The GridSearchCV course of evaluated 25 totally different combos throughout 5 folds, totaling 125 matches:
Becoming 5 folds for every of 25 candidates, totalling 125 matches Finest parameters (Grid Search): {‘regressor__learning_rate’: 0.1, ‘regressor__n_estimators’: 500} Finest rating (Grid Search): 0.9089 |
It confirmed {that a} learning_rate
of 0.1—the default setting—stays efficient. Nonetheless, it steered a rise to 500 timber may barely enhance our mannequin’s efficiency, elevating the R² rating to 0.9089. This can be a modest enhancement in comparison with the R² of 0.9061 achieved earlier with 200 timber and a learning_rate
of 0.1. Curiously, our earlier randomized search yielded a good higher results of 0.9134 with solely 200 timber and learning_rate
roughly 0.158, illustrating the potential advantages of exploring a broader parameter house to optimize efficiency.
To make sure that we have now totally explored the parameter house and to uncover even higher configurations doubtlessly, we’ll now make use of RandomizedSearchCV. This methodology permits for a extra explorative and fewer deterministic method by sampling from a steady distribution of parameter values.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
# Construct on earlier blocks of code from scipy.stats import uniform, randint
# Parameter distribution for RandomizedSearchCV param_dist = { ‘regressor__learning_rate’: uniform(0.001, 0.299), # Uniform distribution between 0.001 and 0.3 ‘regressor__n_estimators’: randint(100, 501) # Uniform distribution of integers from 100 to 500 }
# Setup the RandomizedSearchCV random_search = RandomizedSearchCV(model_pipeline, param_distributions=param_dist, n_iter=50, cv=5, scoring=‘r2’, verbose=1, random_state=42)
# Match the RandomizedSearchCV to the info random_search.match(Ames.drop(columns=‘SalePrice’), Ames[‘SalePrice’])
# Finest parameters and greatest rating from Random Search print(“Finest parameters (Random Search):”, random_search.best_params_) print(“Finest rating (Random Search):”, spherical((random_search.best_score_), 4)) |
The RandomizedSearchCV prolonged our search throughout a broader vary of potentialities, testing 50 totally different configurations throughout 5 folds, totaling 250 matches:
Becoming 5 folds for every of 50 candidates, totalling 250 matches Finest parameters (Random Search): {‘regressor__learning_rate’: 0.12055843054286139, ‘regressor__n_estimators’: 287} Finest rating (Random Search): 0.9158 |
It recognized an much more efficient setting with a learning_rate
of roughly 0.121 and n_estimators
at 287, attaining our greatest R² rating but at 0.9158. This underscores the potential of randomized parameter tuning to find optimum settings that extra inflexible strategies would possibly miss.
To validate the efficiency enhancements achieved by way of our tuning efforts, we are going to now carry out a ultimate cross-validation utilizing the Gradient Boosting Regressor configured with the very best parameters recognized: n_estimators
set to 287 and a learning_rate
of roughly 0.121.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
# Construct on earlier blocks of code # Cross verify mannequin efficiency of Gradient Boosting Regressor with tuned parameters
# ‘preprocessor’ is already arrange as your preprocessing pipeline model_pipeline = Pipeline([ (‘preprocessor’, preprocessor), (‘regressor’, GradientBoostingRegressor(n_estimators=287, learning_rate=0.12055843054286139, random_state=42)) ])
# Utilizing the complete dataset X, y X = Ames.drop(columns=‘SalePrice’) y = Ames[‘SalePrice’]
# Carry out 5-fold cross-validation cv_scores = cross_val_score(model_pipeline, X, y, cv=5, scoring=‘r2’)
# Output the imply cross-validated rating of tuned mannequin print(“Efficiency of Gradient Boosting Regressor with tuned parameters:”, spherical(cv_scores.imply(), 4)) |
The ultimate output confirms the efficiency of our tuned Gradient Boosting Regressor.
Efficiency of Gradient Boosting Regressor with tuned parameters: 0.9158 |
By optimizing each learning_rate
and n_estimators
, we have now achieved an R² rating of 0.9158. This rating not solely validates the enhancements made by way of parameter tuning but in addition emphasizes the aptitude of the Gradient Boosting Regressor to adapt and carry out constantly throughout the dataset.
APIs
Tutorials
Ames Housing Dataset & Information Dictionary
Abstract
This publish explored the capabilities of the Gradient Boosting Regressor (GBR), from understanding the foundational ideas of boosting to superior optimization methods utilizing the Ames Housing Dataset. It centered on key parameters of the GBR such because the variety of timber and studying price, important for refining the mannequin’s accuracy and effectivity. By way of systematic and randomized approaches, it demonstrated the best way to fine-tune these parameters utilizing GridSearchCV and RandomizedSearchCV, enhancing the mannequin’s efficiency considerably.
Particularly, you discovered:
- The basics of boosting and the way it differs from different ensemble methods like bagging.
- Learn how to obtain incremental enhancements by experimenting with a spread of fashions.
- Methods for tuning studying price and variety of timber for the Gradient Boosting Regressor.
Do you might have any questions? Please ask your questions within the feedback under, and I’ll do my greatest to reply.