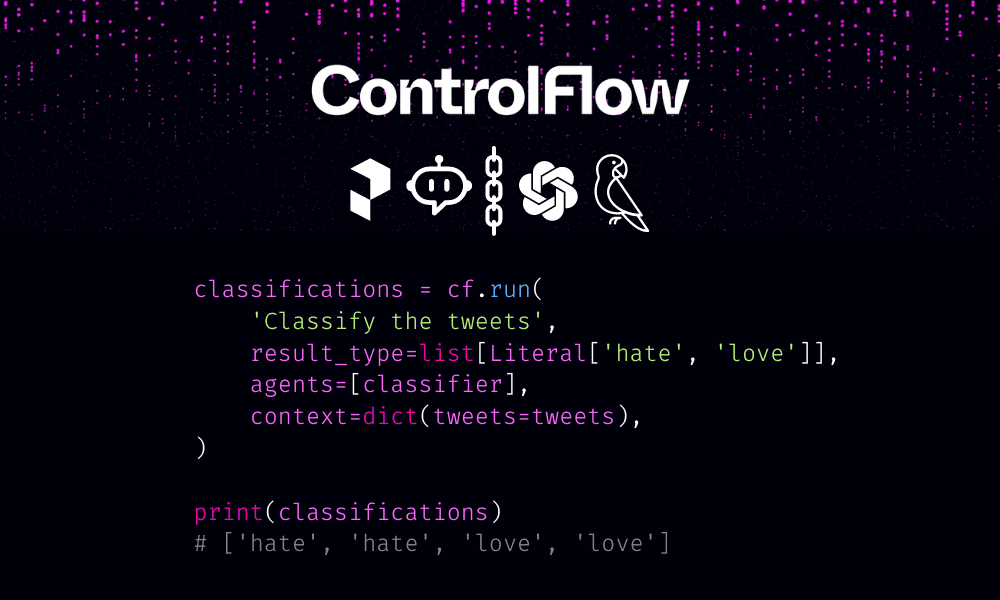
Constructing 3 Enjoyable AI Functions with ControlFlow
Picture by Creator | Canva Professional
The AI business is quickly advancing in direction of creating options utilizing massive language fashions (LLMs) and maximizing the potential of AI fashions. Corporations are in search of instruments that seamlessly combine AI into present codebases with out the hefty prices related to hiring professionals and buying sources. That is the place Controlflow comes into play. With ControlFlow, you possibly can develop complicated AI purposes utilizing only a few traces of code.
On this tutorial, we’ll discover ControlFlow and use it to construct three thrilling AI purposes. The initiatives vary from a easy textual content classifier to complicated AI with a number of brokers, duties, and stream.
What’s ControlFlow?
ControlFlow is a Python framework that gives a structured method for outlining LLM workflows. It consists of three foremost parts for creating AI purposes:
- Duties: These are the basic constructing blocks of AI workflows. They outline discrete, well-defined goals that should be completed by a number of AI brokers.
- Brokers: These are the clever, autonomous entities powering your AI workflows. You’ll be able to outline a mannequin, present it with customized directions, and add varied instruments to create the brokers.
- Flows: Flows are liable for working a number of AI workflows in a specified order. They provide a structured approach to handle duties, brokers, instruments, and shared contexts.
Through the use of ControlFlow, you possibly can seamlessly combine AI capabilities into your Python purposes, achieve extra management over AI workflows, and generate structured outputs somewhat than simply textual content. It lets you construct complicated workflows with ease and is extremely user-friendly. The most effective a part of utilizing ControlFlow is that it lets you observe the AI mannequin’s decision-making course of at each activity.
In easy phrases, ControlFlow has two vital makes use of: it orchestrates your LLM workflows and helps you generate structured outputs, providing you with extra management over AI.
Establishing ControlFlow
We will merely set up ControlFlow by typing the next command within the terminal. It would routinely set up all of the dependencies.
$ pip set up controlflow |
Generate the OpenAI API key and set it as an atmosphere variable.
$ export OPENAI_API_KEY=“your-api-key” |
Earlier than utilizing ControlFlow, guarantee it’s correctly put in. Sort the next command within the terminal to view all Python package deal variations related to ControlFlow.
Output:
ControlFlow model: 0.9.4 Prefect model: 3.0.2 LangChain Core model: 0.2.40 Python model: 3.10.12 Platform: Linux–6.1.85+–x86_64–<b>with</b>–glibc2.35 Path: /usr/native/lib/python3.10 |
Creating an agent and working the duty is kind of easy in ControlFlow. On this instance, we have now created the Horror storytelling agent by offering it with customized directions. Then, we’ll use it to run a easy activity by offering it with a immediate. Finally, we’re producing a brief story.
import controlflow as cf
teller = cf.Agent(title=“Horror Storyteller”, mannequin=“openai/gpt-4o”, directions=“You might be an older man telling horror tales to youngsters.”)
story = cf.run(“Write a brief story.”, brokers=[teller]) |
Right here is the outcome.
In case you encounter a RuntimeError
or runtime situation whereas working the next code in Colab, please rerun the cell as soon as once more.
1. Tweet Classification
Tweet Classifier is a well-liked small challenge for college students, and it often takes them months to construct a correct textual content classifier. Through the use of ControlFlow, we are able to create a correct tweet classifier utilizing just a few traces of code.
- Create a listing of 4 quick tweets.
- Setup an agent with customized directions utilizing the GPT-4-mini mannequin.
import controlflow as cf
tweets = [ “Negativity spreads too easily here. #sigh”, “Sometimes venting is necessary. #HateTherapy”, “Love fills the air today! 💖 #Blessed”, “Thankful for all my Twitter friends! 🌟” ] # Create a specialised agent classifier = cf.Agent( title=“Tweet Classifier”, mannequin=“openai/gpt-4o-mini”, directions=“You might be an professional at rapidly classifying tweets.”, ) |
- Create a activity to categorise tweets as “hate” or “love”, with a immediate, outcome sort, brokers, and context. We are going to present a listing of tweets as context.
- Run the duty and show the outcomes.
from typing import Literal
# Arrange a ControlFlow activity to categorise tweets classifications = cf.run( ‘Classify the tweets’, result_type=record[Literal[‘hate’, ‘love’]], brokers=[classifier], context=dict(tweets=tweets), )
print(classifications) |
ControlFlow duties have categorised tweets and generated a listing of labels.
Let’s show them in a correct manner utilizing the colour code (crimson for hate and inexperienced for love).