Introduction
Arrays in Python are knowledge constructions that may maintain a number of values of the identical knowledge sort. They supply a approach to retailer and manipulate collections of information effectively. On this article, we are going to discover how you can work with arrays in Python, together with creating arrays, accessing and manipulating array parts, performing array operations, working with multi-dimensional arrays, and utilizing arrays in real-world purposes.
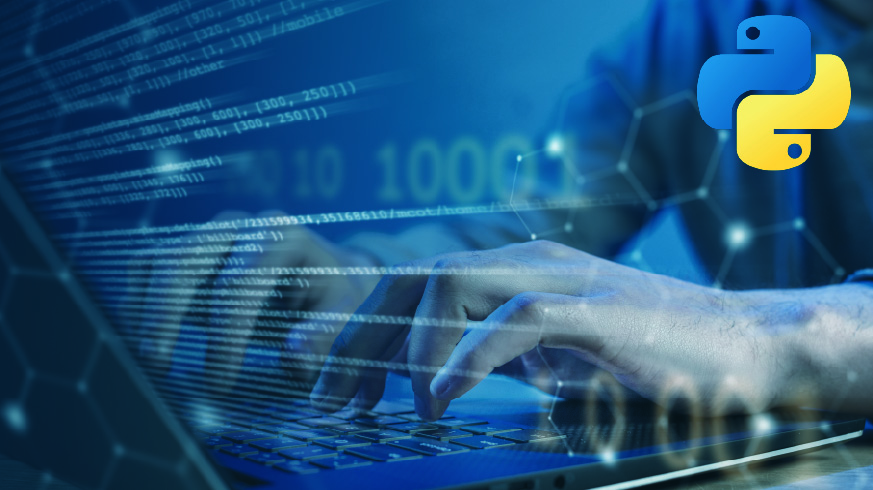
Creating Arrays in Python
There are a number of methods to create arrays in Python. One widespread methodology is utilizing the NumPy library. NumPy helps multi-dimensional arrays and varied mathematical features for array manipulation. Right here’s how one can create a NumPy array:
import numpy as np
my_array = np.array([1, 2, 3, 4, 5])
sort(my_array)
Output: numpy.ndarray
You may also create arrays utilizing the Array module in Python, which offers extra functionalities for array manipulation.
import array
# Create an array of integers
int_array = array.array('i', [1, 2, 3, 4, 5])
sort(int_array)
Output: array.array
Accessing and Manipulating Arrays
Upon getting created an array, you may entry and manipulate its parts utilizing indexing and slicing. Indexing means that you can entry particular parts within the array, whereas slicing allows you to extract a subset of parts. Right here’s an instance:
my_array = np.array([1, 2, 3, 4, 5])
# Accessing parts
print(my_array[0]) # Output: 1
# Slicing
print(my_array[1:4])
Output: [2, 3, 4]
You may also add and take away parts from an array and replace current parts to change the array’s content material.
# Including parts
my_array = np.append(my_array, 6)
print("After including a component:", my_array) # Output: [1 2 3 4 5 6]
# Eradicating parts
my_array = np.delete(my_array, 2) # Take away aspect at index 2
print("After eradicating a component:", my_array) # Output: [1 2 4 5 6]
# Updating parts
my_array[3] = 7
print("After updating a component:", my_array)
Output: [1 2 4 7 6]
Array Operations and Features
Arrays in Python assist varied operations and features for environment friendly knowledge manipulation. You may concatenate arrays, reshape their dimensions, type parts, and seek for particular values inside an array. These operations are important for performing complicated knowledge processing duties.
# Array Concatenation
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
concatenated_array = np.concatenate((array1, array2))
print(concatenated_array) # Output: [1 2 3 4 5 6]
# Array Reshaping
reshaped_array = concatenated_array.reshape(2, 3)
print(reshaped_array)
Output: [[1 2 3]
[4 5 6]]
# Array Sorting
sorted_array = np.type(concatenated_array)
print(sorted_array) # Output: [1 2 3 4 5 6]
# Array Looking
index = np.the place(sorted_array == 3)
print(index)
Output: (array([2]),)
Multi-dimensional Arrays
Along with one-dimensional arrays, Python additionally helps multi-dimensional arrays utilizing NumPy. Multi-dimensional arrays signify complicated knowledge constructions equivalent to matrices and tensors. Right here’s an instance of making and accessing a multi-dimensional array:
multi_array = np.array([[1, 2, 3], [4, 5, 6]])
print(multi_array)
Output: [[1 2 3]
[4 5 6]]
You may carry out varied operations on multi-dimensional arrays, equivalent to element-wise arithmetic operations, matrix multiplication, and transposition.
Additionally learn: Features 101 – Introduction to Features in Python For Absolute Freshmen.
Comparability of Numpy Array Methodology and Array Module
- NumPy Array Methodology
- Provides intensive performance for numerical computing.
- Helps multidimensional arrays and various knowledge varieties.
- Optimized for environment friendly mathematical operations.
- Extensively utilized in scientific computing and knowledge evaluation.
- Supplies wealthy array manipulation and broadcasting.
- Most well-liked for complicated duties requiring superior functionalities.
- Array Module
- Supplies a less complicated various to primary array manipulation.
- Arrays are one-dimensional and homogeneous.
- Provides restricted knowledge varieties and primary operations.
- Extra reminiscence environment friendly in comparison with lists for big datasets.
- Appropriate for easy duties with homogeneous knowledge.
- Lacks superior options and optimizations of NumPy arrays.
Working with Arrays in Actual-world Purposes
Arrays are essential in varied real-world purposes, together with knowledge evaluation and visualization, machine studying and AI, and scientific computing. They supply a basis for dealing with massive datasets, performing complicated calculations, and implementing algorithms effectively.
Finest Practices for Utilizing Arrays in Python
When working with arrays in Python, it’s important to comply with finest practices for environment friendly reminiscence administration, choosing the proper knowledge construction, and optimizing array operations. By optimizing your code and knowledge constructions, you may enhance efficiency and scalability in your purposes.
Conclusion
In conclusion, arrays are highly effective knowledge constructions in Python that allow you to retailer, manipulate, and analyze knowledge collections successfully. By understanding how you can create arrays, entry and manipulate array parts, carry out array operations, and work with multi-dimensional arrays, you may leverage the complete potential of arrays in your Python initiatives.
By following finest practices and exploring the huge array of functionalities obtainable in Python libraries equivalent to NumPy, you may simply take your array manipulation abilities to the subsequent stage and sort out complicated knowledge processing duties. Begin experimenting with arrays in Python immediately and unlock a world of potentialities in knowledge science, machine studying, and scientific computing.
In case you are in search of a web-based Python course, try this “Study Python for Knowledge Science.”