With regards to designing visually interesting and intuitive person interfaces in iOS growth, SF Symbols are a useful asset. It affords a complete library of over 5,000 customizable icons, designed particularly for iOS and macOS purposes. The newest iOS 17 replace brings SF Symbols 5, which introduces a implausible assortment of expressive animations. SwiftUI affords builders the flexibility to leverage these animations utilizing the brand new symbolEffect
modifier.
This function empowers builders to create various and fascinating animations inside their apps. By incorporating symbolEffect
into your SwiftUI code, builders can improve person interactions and create visually partaking interfaces. On this tutorial, we are going to present you the best way to work with this new modifier to create numerous kinds of animations.
The Fundamental Utilization of SymbolEffect
To animate a SF image, you’ll be able to connect the brand new symbolEffect
modifier to the Picture
view and specify the specified animation kind. Right here is an instance:
var physique: some View {
Picture(systemName: “ellipsis.message”)
.font(.system(measurement: 100))
.symbolRenderingMode(.palette)
.foregroundStyle(.purple, .grey)
.symbolEffect(.bounce, worth: animate)
.onTapGesture {
animate.toggle()
}
}
}
struct ContentView: View { @State personal var animate = false
var physique: some View { Picture(systemName: “ellipsis.message”) .font(.system(measurement: 100)) .symbolRenderingMode(.palette) .foregroundStyle(.purple, .grey) .symbolEffect(.bounce, worth: animate) .onTapGesture { animate.toggle() } } } |
There are a variety of built-in animations together with Seem, Disappear, Bounce, Scale, Pulse, Variable Shade, and Change. Within the code above, we use the bounce
animation. So, if you faucet the image within the preview canvas, it reveals a bouncing impact.
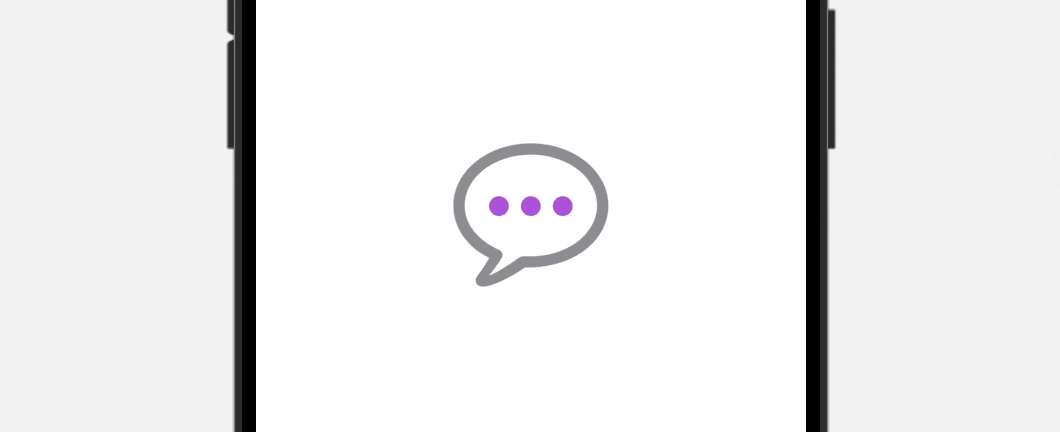
Make it Repeatable
By default, the animation is simply performed as soon as. To make it repeatable, you’ll be able to set the choices
parameter of the modifier to .repeating
like this:
.symbolEffect(.bounce, choices: .repeating, worth: animate) |
This can obtain an animated impact that repeats indefinitely. In the event you need to repeat the impact for a particular variety of occasions, you’ll be able to make the most of the .repeat
operate and point out the specified repeat rely as proven under:
.symbolEffect(.bounce, choices: .repeat(5), worth: animate) |
Controlling the animation pace
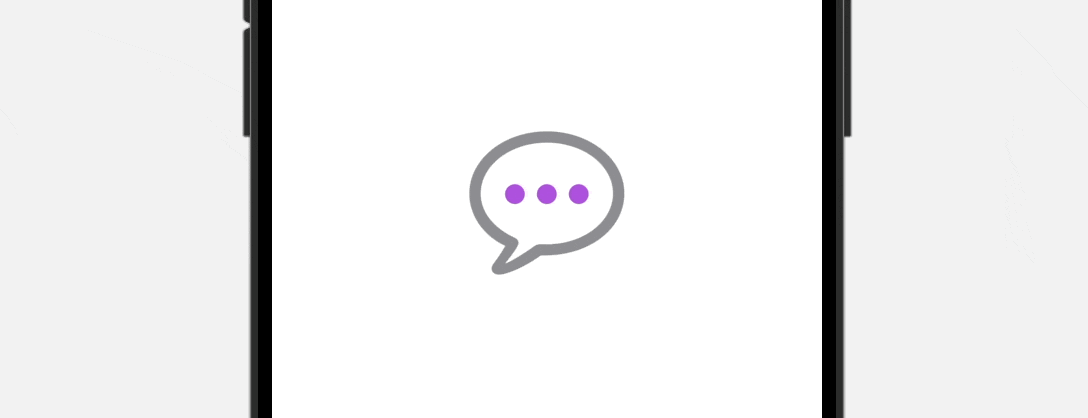
As well as, you could have the flexibleness to customise the animation pace by using the .pace
operate inside the choices parameter. As an illustration, for those who want to decelerate the animation, you’ll be able to set the worth of the .pace
operate to 0.1, as demonstrated under:
.symbolEffect(.bounce, choices: .pace(0.1), worth: animate) |
Animation Varieties
As said earlier, SwiftUI gives quite a lot of built-in animation sorts, reminiscent of Bounce, Scale, Pulse, Variable Shade, and Change. Up till now, we have now solely used the bounce animation. Now, let’s discover and take a look at out different animation sorts utilizing the offered code snippet:
var physique: some View {
VStack(alignment: .main, spacing: 50) {
HStack {
Picture(systemName: “mic.and.sign.meter”)
.font(.system(measurement: 60))
.symbolRenderingMode(.palette)
.foregroundStyle(.purple, .grey)
.symbolEffect(.bounce, choices: .repeating, worth: animate)
Textual content(“Bounce”)
.font(.largeTitle)
}
HStack {
Picture(systemName: “mic.and.sign.meter”)
.font(.system(measurement: 60))
.symbolRenderingMode(.palette)
.foregroundStyle(.purple, .grey)
.symbolEffect(.bounce.down, choices: .repeating, worth: animate)
Textual content(“Bounce (down)”)
.font(.largeTitle)
}
HStack {
Picture(systemName: “mic.and.sign.meter”)
.font(.system(measurement: 60))
.symbolRenderingMode(.palette)
.foregroundStyle(.purple, .grey)
.symbolEffect(.pulse, choices: .repeating, worth: animate)
Textual content(“Pulse”)
.font(.largeTitle)
}
HStack {
Picture(systemName: “mic.and.sign.meter”)
.font(.system(measurement: 60))
.symbolRenderingMode(.palette)
.foregroundStyle(.purple, .grey)
.symbolEffect(.pulse.wholeSymbol, choices: .repeating, worth: animate)
Textual content(“Pulse (complete)”)
.font(.largeTitle)
}
HStack {
Picture(systemName: “mic.and.sign.meter”)
.font(.system(measurement: 60))
.symbolRenderingMode(.palette)
.foregroundStyle(.purple, .grey)
.symbolEffect(.variableColor, choices: .repeating, worth: animate)
Textual content(“Variable colour”)
.font(.largeTitle)
}
}
.onTapGesture {
animate.toggle()
}
}
}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
struct SymbolAnimationView: View { @State personal var animate = false
var physique: some View { VStack(alignment: .main, spacing: 50) { HStack { Picture(systemName: “mic.and.sign.meter”) .font(.system(measurement: 60)) .symbolRenderingMode(.palette) .foregroundStyle(.purple, .grey) .symbolEffect(.bounce, choices: .repeating, worth: animate) Textual content(“Bounce”) .font(.largeTitle) }
HStack { Picture(systemName: “mic.and.sign.meter”) .font(.system(measurement: 60)) .symbolRenderingMode(.palette) .foregroundStyle(.purple, .grey) .symbolEffect(.bounce.down, choices: .repeating, worth: animate) Textual content(“Bounce (down)”) .font(.largeTitle) }
HStack { Picture(systemName: “mic.and.sign.meter”) .font(.system(measurement: 60)) .symbolRenderingMode(.palette) .foregroundStyle(.purple, .grey) .symbolEffect(.pulse, choices: .repeating, worth: animate) Textual content(“Pulse”) .font(.largeTitle) }
HStack { Picture(systemName: “mic.and.sign.meter”) .font(.system(measurement: 60)) .symbolRenderingMode(.palette) .foregroundStyle(.purple, .grey) .symbolEffect(.pulse.wholeSymbol, choices: .repeating, worth: animate) Textual content(“Pulse (complete)”) .font(.largeTitle) }
HStack { Picture(systemName: “mic.and.sign.meter”) .font(.system(measurement: 60)) .symbolRenderingMode(.palette) .foregroundStyle(.purple, .grey) .symbolEffect(.variableColor, choices: .repeating, worth: animate) Textual content(“Variable colour”) .font(.largeTitle) }
} .onTapGesture { animate.toggle() } } } |
By tapping any of the photographs within the preview canvas, you’ll be able to see the animations coming to life. Compared to the bounce animation, the Pulse animation affords a definite impact by steadily fading the opacity of particular or all layers inside the picture. Alternatively, the variableColor
animation replaces the opacity of variable layers within the picture, offering a singular visible transformation.

Even for the Bounce animation, you’ll be able to specify .bounce.down
to bounce the image downward.
.symbolEffect(.bounce.down, choices: .repeating, worth: animate) |
For added flexibility, it’s attainable to use a number of symbolEffect modifiers to a view, permitting you to realize a customized impact by combining completely different animations.
Picture(systemName: “ellipsis.message”) .font(.system(measurement: 100)) .symbolRenderingMode(.palette) .foregroundStyle(.purple, .grey) .symbolEffect(.bounce, choices: .pace(1.5), worth: animate) .symbolEffect(.pulse, choices: .repeating, worth: animate) .onTapGesture { animate.toggle() } |
Content material Transition and Change Animation
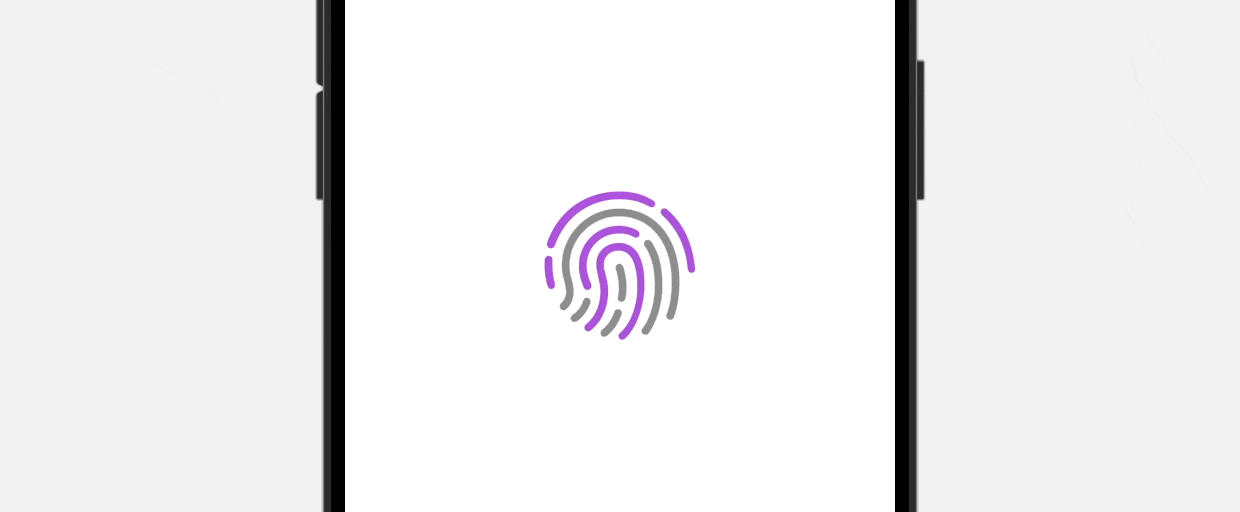
In sure situations, there could also be a must transition between completely different symbols inside a picture. As an illustration, when a person faucets the Contact ID image, it transforms right into a checkmark image. To make sure a seamless and visually pleasing transition, you’ll be able to make the most of the contentTransition
modifier at the side of the Change
animation, as demonstrated under:
Picture(systemName: animate ? “checkmark.circle” : “touchid”) .font(.system(measurement: 100)) .symbolRenderingMode(.palette) .symbolEffect(.bounce, worth: animate) .contentTransition(.symbolEffect(.change)) .foregroundStyle(.purple, .grey) .onTapGesture { animate.toggle() } |
Abstract
SF Symbols and symbolEffect
present builders with highly effective instruments to boost person interactions and create visually partaking interfaces in iOS and macOS purposes.
This tutorial demonstrates the essential utilization of symbolEffect
, making animations repeatable, controlling animation pace, and exploring completely different animation sorts. It additionally covers content material transition and change animation.
When you have discovered this tutorial pleasurable and want to discover SwiftUI additional, we extremely suggest trying out our complete guide, “Mastering SwiftUI.“