In SwiftUI, there’s a handy built-in modifier known as cornerRadius
that means that you can simply create rounded corners for a view. By making use of the cornerRadius
modifier to a Rectangle
view, you may rework it right into a rounded rectangle. The worth you present to the modifier determines the extent of the rounding impact.
Rectangle() .cornerRadius(10.0) |
Alternatively, SwiftUI additionally supplies a normal RoundedRectangle
view for making a rounded rectangle:
RoundedRectangle(cornerRadius: 25.0) |
Sadly, each the cornerRadius
modifier and the RoundedRectangle
view can solely apply the identical nook radius to all corners of the form.
What if you have to spherical a particular nook of a view?
In iOS 17, the SwiftUI framework introduces a brand new view known as UnevenRoundedRectangle
. What units this view aside is the flexibility to specify a definite radius worth for every nook, permitting builders to create extremely customizable shapes.
Notice: We have now a tutorial on UIKit exhibiting you how one can spherical particular corners.
Working with UnevenRoundedRectangle
With UnevenRoundedRectangle
, you may simply create rectangular shapes with rounded corners of various radii. To make use of UnevenRoundedRectangle
, you merely must specify the nook radius for every nook. Right here is an instance:
UnevenRoundedRectangle(cornerRadii: .init( topLeading: 50.0, bottomLeading: 10.0, bottomTrailing: 50.0, topTrailing: 30.0), model: .steady) .body(width: 300, peak: 100) .foregroundStyle(.indigo) |
Optionally, you may point out the model of the corners. A steady
nook model will give the corners a smoother look. In the event you’ve put the code above in Xcode 15, you may create an oblong form like under.
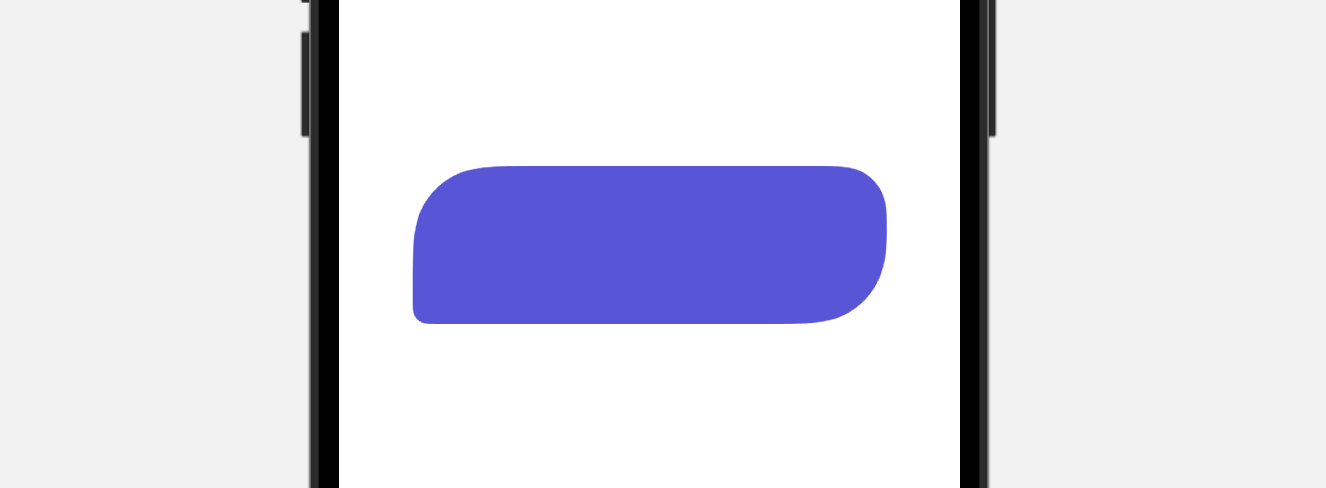
You should use this form and rework it right into a button through the use of the background
modifier. Here’s a pattern code snippet:
}) {
Textual content(“Register”)
.font(.title)
}
.tint(.white)
.body(width: 300, peak: 100)
.background {
UnevenRoundedRectangle(cornerRadii: .init(
topLeading: 50.0,
bottomLeading: 10.0,
bottomTrailing: 50.0,
topTrailing: 30.0),
model: .steady)
.foregroundStyle(.indigo)
}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
Button(motion: {
}) { Textual content(“Register”) .font(.title) } .tint(.white) .body(width: 300, peak: 100) .background { UnevenRoundedRectangle(cornerRadii: .init( topLeading: 50.0, bottomLeading: 10.0, bottomTrailing: 50.0, topTrailing: 30.0), model: .steady) .foregroundStyle(.indigo) } |
Animating the Rounded Corners

To animate the rounded corners of the UnevenRoundedRectangle
, you need to use the withAnimation
perform and toggle a Boolean variable. Right here is an instance code snippet:
@State personal var animate = false
var physique: some View {
UnevenRoundedRectangle(cornerRadii: .init(
topLeading: animate ? 10.0 : 80.0,
bottomLeading: animate ? 80.0 : 10.0,
bottomTrailing: animate ? 80.0 : 10.0,
topTrailing: animate ? 10.0 : 80.0))
.foregroundStyle(.indigo)
.body(peak: 200)
.padding()
.onTapGesture {
withAnimation {
animate.toggle()
}
}
}
}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
struct AnimatedCornerView: View {
@State personal var animate = false
var physique: some View {
UnevenRoundedRectangle(cornerRadii: .init( topLeading: animate ? 10.0 : 80.0, bottomLeading: animate ? 80.0 : 10.0, bottomTrailing: animate ? 80.0 : 10.0, topTrailing: animate ? 10.0 : 80.0)) .foregroundStyle(.indigo) .body(peak: 200) .padding() .onTapGesture { withAnimation { animate.toggle() } }
} } |
On this instance, tapping the rectangle will toggle the animate
variable, which controls the nook radii of the rectangle. The withAnimation
perform will animate the transition between the 2 units of nook radii.
Creating Distinctive Shapes
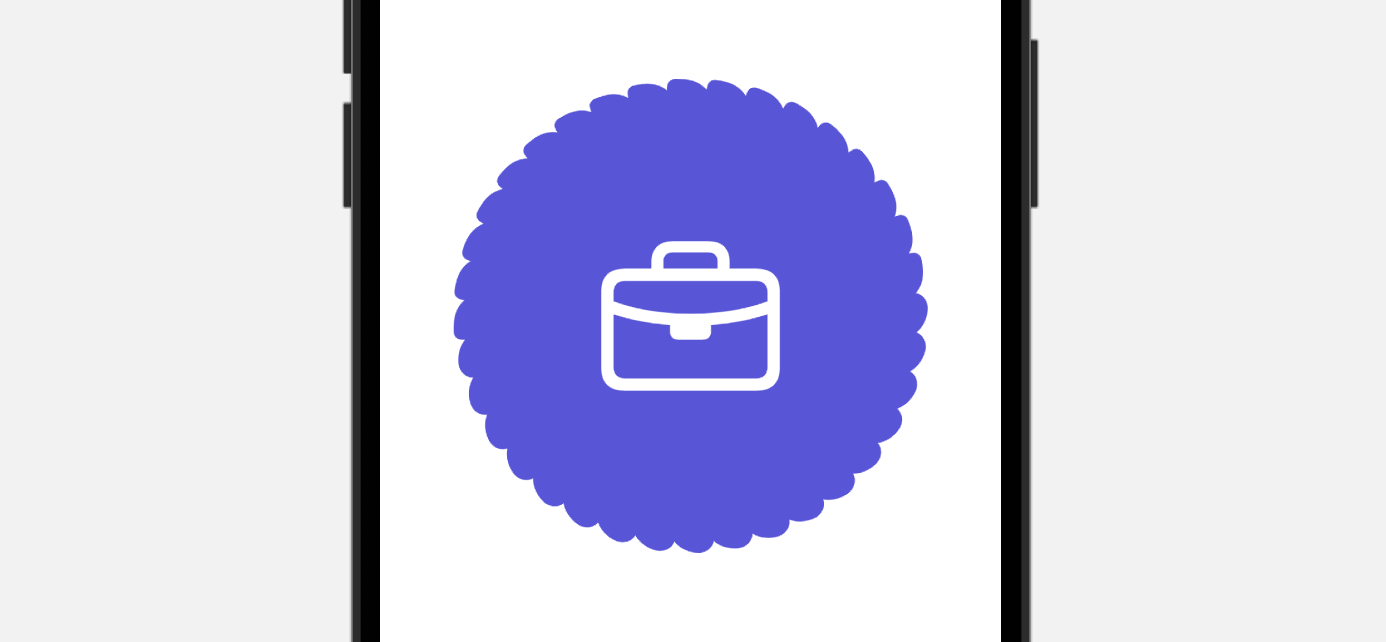
By overlapping a number of UnevenRoundedRectangle
views, you may create all kinds of shapes. The instance offered above demonstrates how one can create the particular form proven utilizing the next code:
ZStack { ForEach(0..<18, id: .self) { index in UnevenRoundedRectangle(cornerRadii: .init(topLeading: 20.0, bottomLeading: 5.0, bottomTrailing: 20.0, topTrailing: 10.0), model: .steady) .foregroundStyle(.indigo) .body(width: 300, peak: 30) .rotationEffect(.levels(Double(10 * index))) } } .overlay { Picture(systemName: “briefcase”) .foregroundStyle(.white) .font(.system(measurement: 100)) } |
So as to add an extra visible impact, you may animate the change in opacity by modifying the code as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
ZStack { ForEach(0..<18, id: .self) { index in UnevenRoundedRectangle(cornerRadii: .init(topLeading: 20.0, bottomLeading: 5.0, bottomTrailing: 20.0, topTrailing: 10.0), model: .steady) .foregroundStyle(.indigo) .body(width: 300, peak: 30) .opacity(animate ? 0.6 : 1.0) .rotationEffect(.levels(Double(10 * index))) .animation(.easeInOut.delay(Double(index) * 0.02), worth: animate) } } .overlay { Picture(systemName: “briefcase”) .foregroundStyle(.white) .font(.system(measurement: 100)) } .onTapGesture { animate.toggle() } |
Implementing this modification will lead to a visually fascinating impact that provides an additional stage of curiosity to your design.
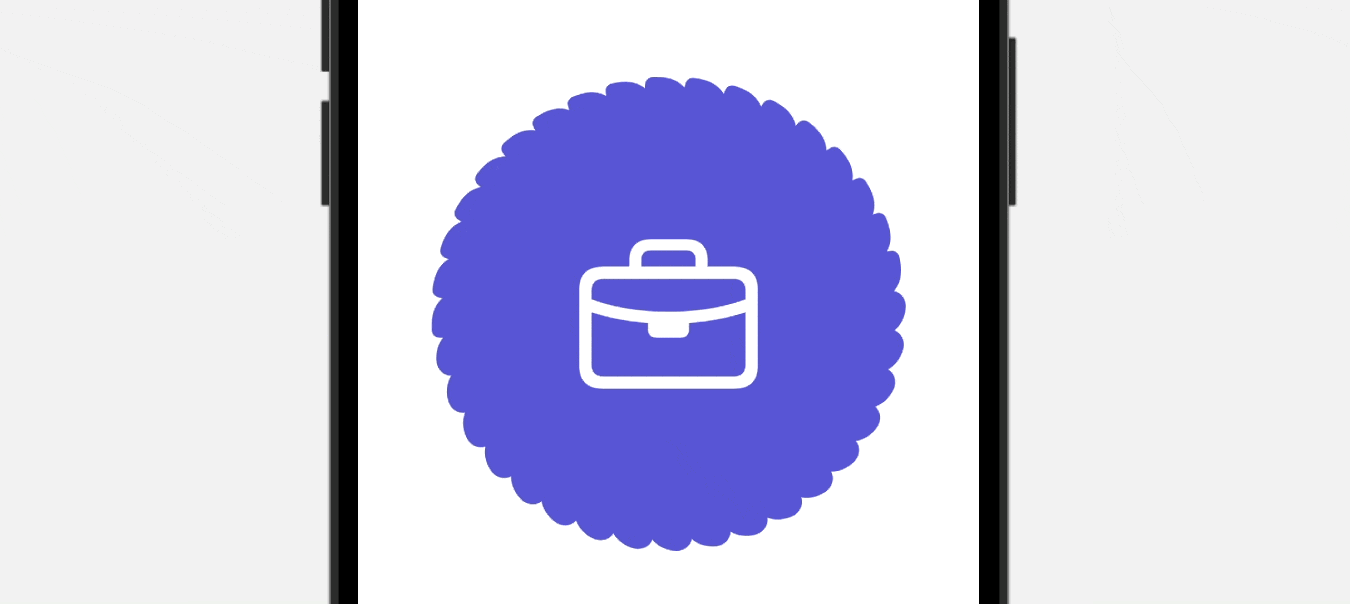
Abstract
The addition of the UnevenRoundedRectangle
view in SwiftUI supplies builders with a handy resolution for rounding particular corners of rectangular views. It additionally provides you the pliability and choices to attain the look you need. With the UnevenRoundedRectangle
form, you may seamlessly incorporate distinctive and crowd pleasing shapes into your app, enhancing its total design and person expertise.